Learn how to use Amazon S3 endpoints to securely access data from an EC2 instance located in a private subnet.
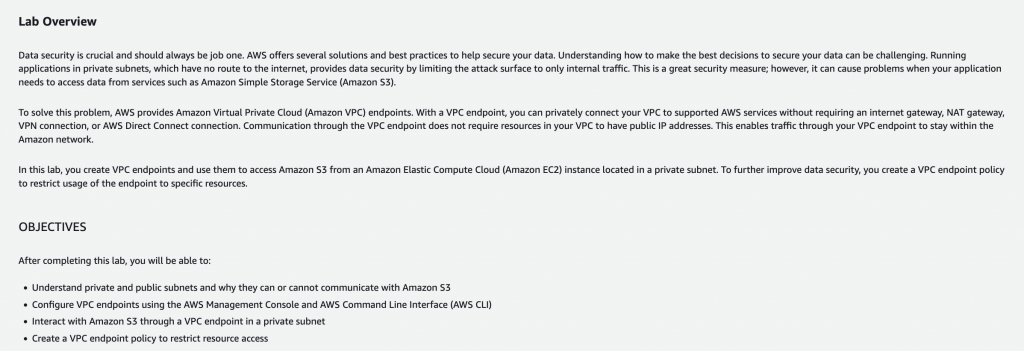
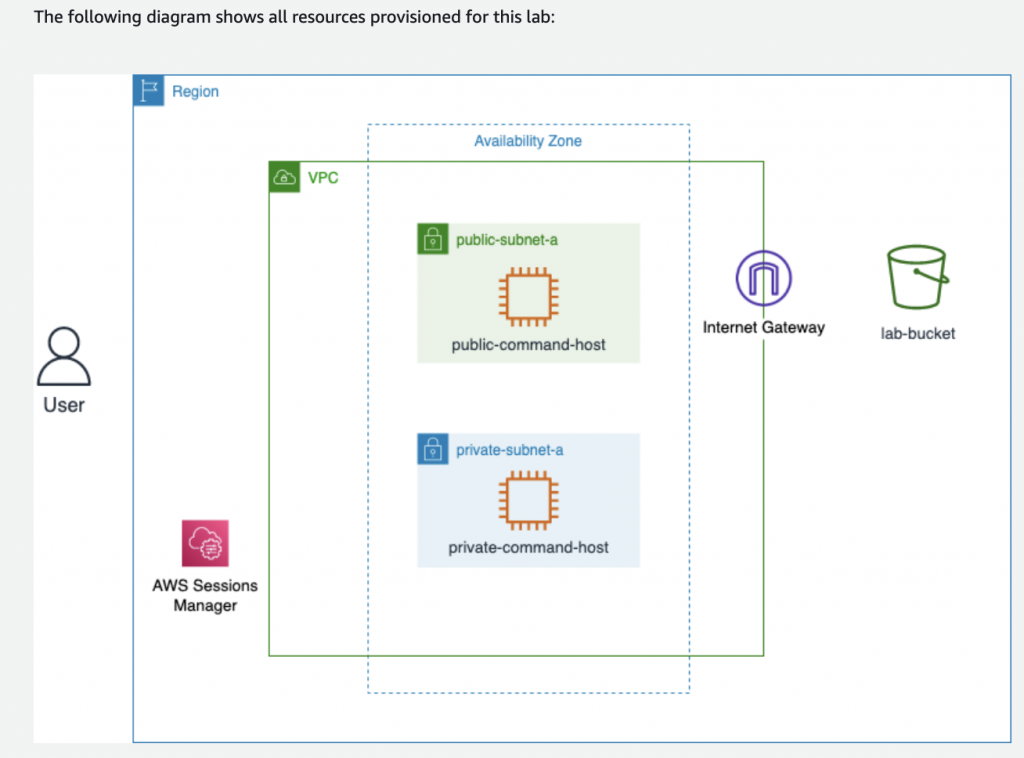
ls
// To go to your home directory, run the following command
cd ~
// To help differentiate commands from output in the AWS CLI, run the following command. This adds a blank line before any output to the screen:
trap 'printf "\n"' DEBUG
// You can also alter your command prompt to make output easier to read by exporting the PS1 variable. To do this, run the following command:
export PS1="\n[\u@\h \W] $ "
// To configure the AWS CLI, run the following command :
aws configure
rm ~/.aws/credentials
aws s3 ls
aws s3 ls s3://<LabBucket>
aws s3 cp s3://<LabBucket>/demo.txt ~/
less demo.txt
echo "
This is some non-unique text that will be appended to your file." >> demo.txt
less demo.txt
aws s3 cp demo.txt s3://<LabBucket>/
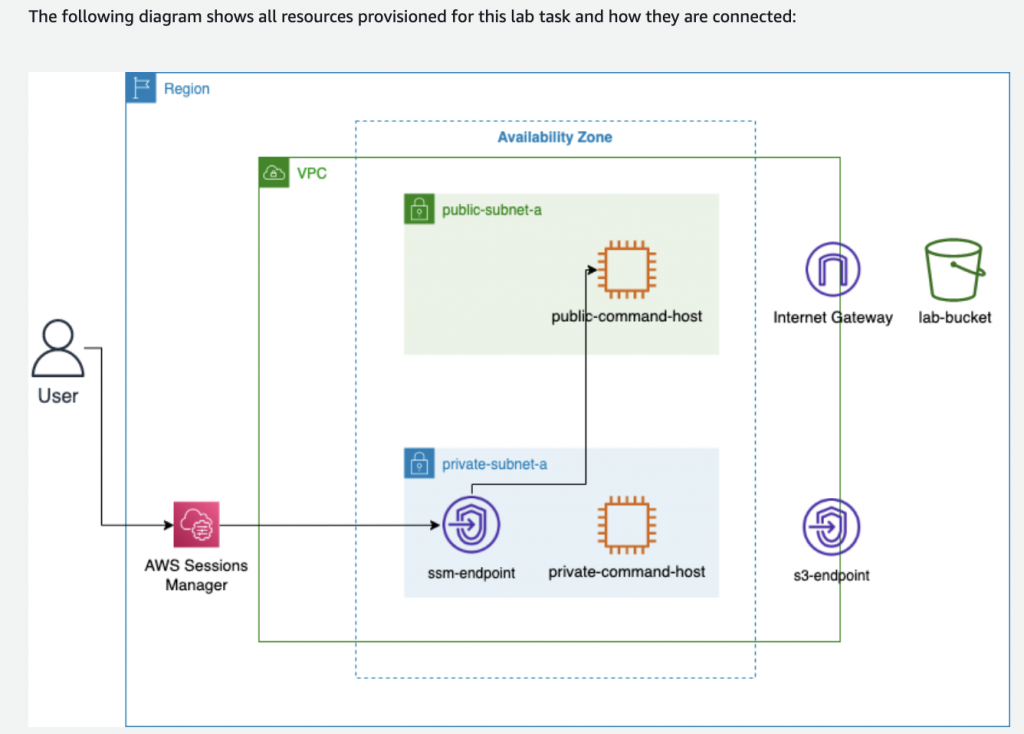
# To list the services that have VPC endpoints created for them, run the following command:
aws ec2 describe-vpc-endpoints --query 'VpcEndpoints[*].ServiceName'
VPC=$(aws ec2 describe-vpcs --query 'Vpcs[*].VpcId' --filters 'Name=tag:Name, Values=labVPC' | jq -r '.[0]')
echo $VPC
RTB=$(aws ec2 describe-route-tables --query 'RouteTables[*].RouteTableId' --filters 'Name=tag:Name, Values=PrivateRouteTable' | jq -r '.[0]')
echo $RTB
export AWS_REGION=$(curl -s 169.254.169.254/latest/dynamic/instance-identity/document | jq -r '.region')
echo $AWS_REGION
aws ec2 create-vpc-endpoint \
--vpc-id $VPC \
--service-name com.amazonaws.$AWS_REGION.s3 \
--route-table-ids $RTB
aws ec2 describe-vpc-endpoints --query 'VpcEndpoints[*].ServiceName'
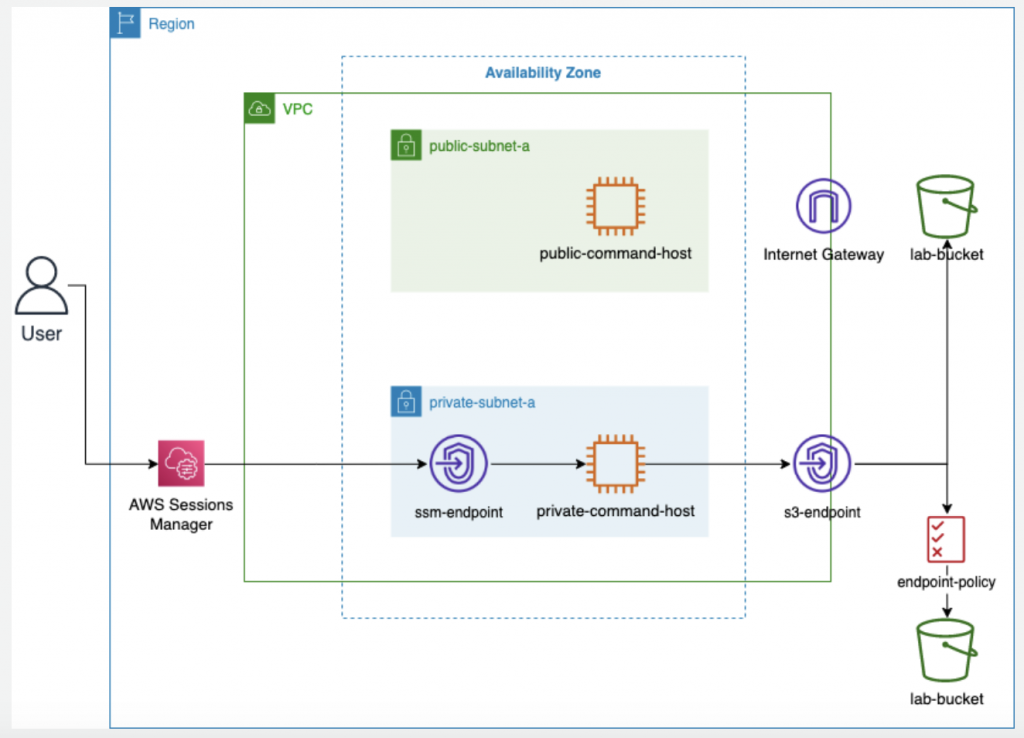
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Principal": "*",
"Action": "s3:List*",
"Resource": "arn:aws:s3:::*"
},
{
"Effect": "Allow",
"Principal": "*",
"Action": "s3:*",
"Resource": [
"arn:aws:s3:::<LabBucket>",
"arn:aws:s3:::<LabBucket>/*"
]
},
{
"Effect": "Deny",
"Principal": "*",
"Action": "s3:*",
"Resource": [
"arn:aws:s3:::<LabLoggingBucket>",
"arn:aws:s3:::<LabLoggingBucket>/*"
]
}
]
}
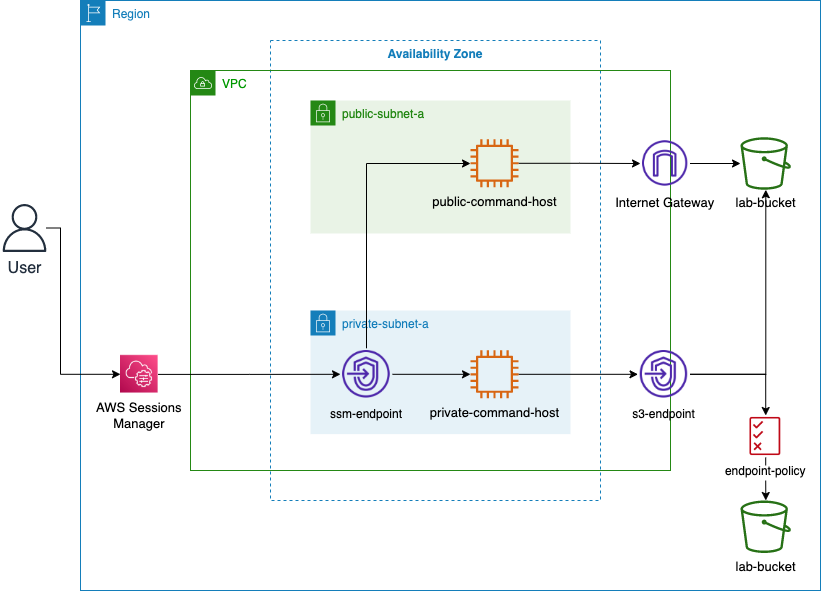
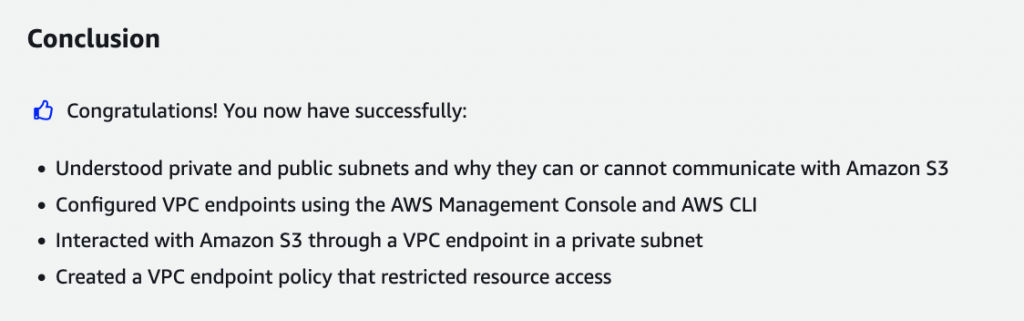
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Principal": "*",
"Action": "s3:List*",
"Resource": "arn:aws:s3:::*"
},
{
"Effect": "Allow",
"Principal": "*",
"Action": "s3:*",
"Resource": [
"arn:aws:s3:::<LabBucket>",
"arn:aws:s3:::<LabBucket>/*"
]
},
{
"Effect": "Deny",
"Principal": "*",
"Action": "s3:*",
"Resource": [
"arn:aws:s3:::<LabLoggingBucket>",
"arn:aws:s3:::<LabLoggingBucket>/*"
]
}
]
}
cd ~
cat <<EOT >> policy.json
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Principal": "*",
"Action": "s3:List*",
"Resource": "arn:aws:s3:::*"
},
{
"Effect": "Allow",
"Principal": "*",
"Action": "s3:*",
"Resource": [
"arn:aws:s3:::<LabBucket>",
"arn:aws:s3:::<LabBucket>/*"
]
},
{
"Effect": "Deny",
"Principal": "*",
"Action": "s3:*",
"Resource": [
"arn:aws:s3:::<LabLoggingBucket>",
"arn:aws:s3:::<LabLoggingBucket>/*"
]
}
]
}
EOT
export vpcEndpointId=$(aws ec2 describe-vpc-endpoints --query 'VpcEndpoints[?contains(ServiceName, `s3`) == `true`].VpcEndpointId' --output text)
echo ${vpcEndpointId}
aws ec2 modify-vpc-endpoint --vpc-endpoint-id ${vpcEndpointId} --policy-document file://policy.json
aws s3 ls s3://<LabBucket>
aws s3 ls s3://<LabLoggingBucket>